NestJs の exceptions layer を利用して、アプリケーション全体で処理されない例外を全て処理する filter を作成します。
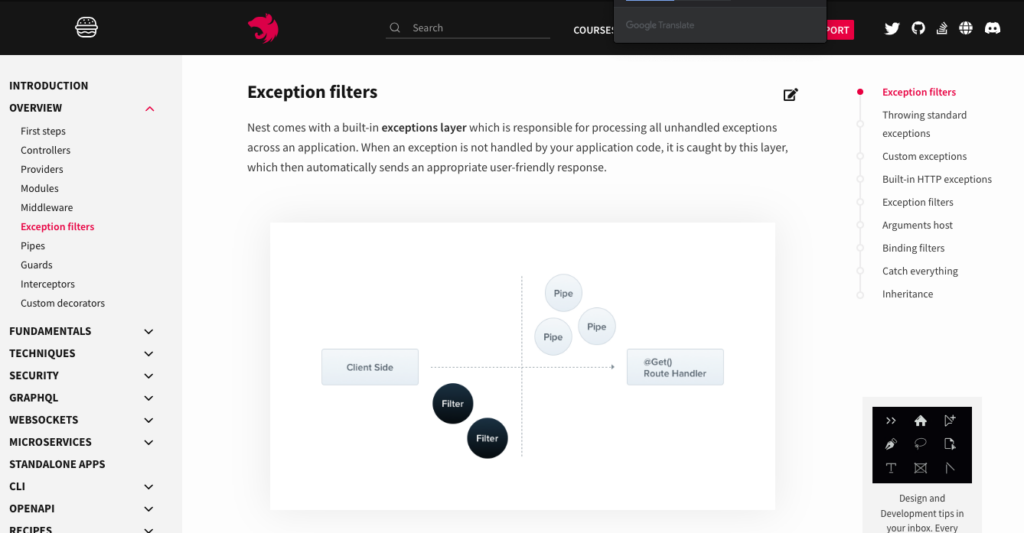
result は空にしてデータを返却します。
{
"status": "false",
"errorCode": "E001",
"result": []
}
目次
エラーコードを定義
constants.ts を定義します。
必要なエラーコードを追加してください。
export default class ErrorCode {
/** 内部サーバーエラー */
static readonly E001 = 'E001';
/** 認証エラー */
static readonly E002 = 'E002';
}
Exception filters を実装
例外フィルターは ExceptionFilter<T> インターフェースを実装する必要があり、@Catch(HttpException) デコレーターをつけることで、例外フィルタに必要なデータがバインドされます。
HttpStatus が500以上の場合は、内部サーバーエラーとして constants.ts の E001 をセットし、それ以外は throw された exception.message をセットします。
import {
ArgumentsHost,
Catch,
ExceptionFilter,
HttpException,
HttpStatus,
} from '@nestjs/common';
import { Response } from 'express';
const responseInterface = {
status: false,
errorCode: '',
result: [],
};
@Catch()
export class HttpExceptionFilter implements ExceptionFilter {
catch(exception: HttpException, host: ArgumentsHost) {
const ctx = host.switchToHttp();
const response = ctx.getResponse<Response>();
const statusCode =
exception instanceof HttpException
? exception.getStatus()
: HttpStatus.INTERNAL_SERVER_ERROR;
responseInterface.status = false;
responseInterface.errorCode = this.setErrorCode(
statusCode,
exception.message,
);
response.status(statusCode).json(responseInterface);
}
private setErrorCode(statusCode: number, message): string {
if (statusCode >= 500) {
return 'E001';
}
return message;
}
}
認証エラーとして、E002を返したい場合は、UnauthorizedException にエラーコードをセットして throw します。
throw new UnauthorizedException(ErrorCode.E002);
Responseの共通化
Response を共通化は、こちらの記事を参照してください。
Tsukurue

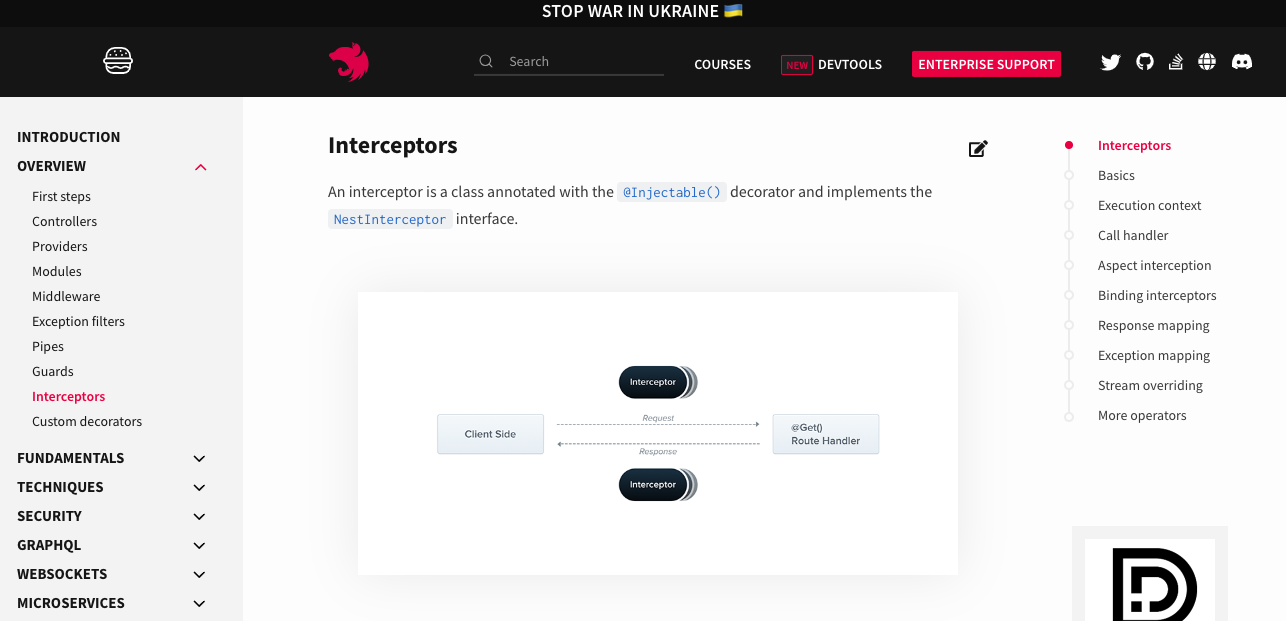
【NestJS】Responseを変換する方法 | Tsukurue
NestJSは、効率的でスケーラブルなNode.jsアプリケーションです。generateコマンドも用意されており、シンプルかつ簡易にAPIを作成することができます。 今回はAPIから返却...