The Web Share API is a mechanism that allows users to share content such as text, links and files to any app of their choice.
This dialogue is displayed when viewed on a smartphone
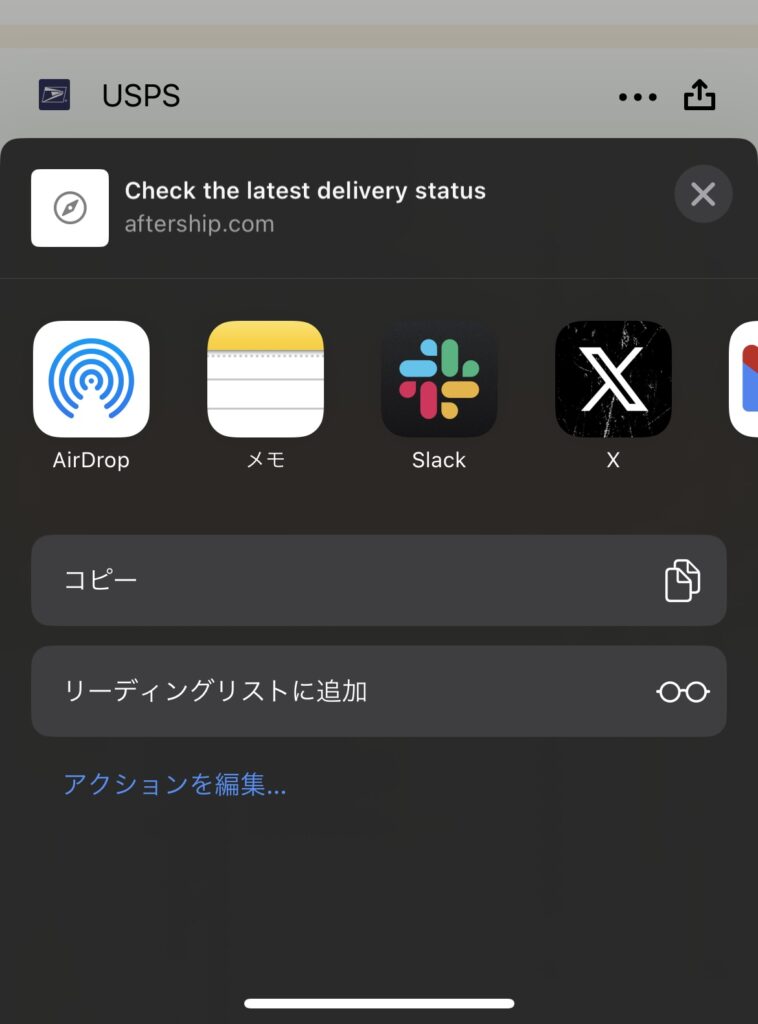
Official website here.
https://developer.mozilla.org/ja/docs/Web/API/Web_Share_API
Implemented Web Share API
The Web Share API is used to raise the content sharing experience.
How to call
The implementation is very simple, just call navigator.share().
const shareData = {
title: "MDN",
text: "Learn web development on MDN!",
url: "https://developer.mozilla.org",
};
await navigator.share(shareData);
Check if the Web Share API can be called.
It is best to check beforehand whether the Web Share API can be called in navigator.canShare.
Check that navigator.share is defined and that the data to be shared can be shared with navigator.canShare.
It can be called when canWebShareApi is true.
const shareData = {
title: "MDN",
text: "Learn web development on MDN!",
url: "https://developer.mozilla.org",
};
const canWebShareApi = !!navigator.share && navigator.canShare?.(shareData);
Official website here.
https://developer.mozilla.org/ja/docs/Web/API/Navigator/canShare
Errors occur when the Web Share API is closed.
This was tricky, but if you close a screen opened with the Web Share API, an error occurs.
Therefore, it should be judged and controlled by error.name.
try {
await navigator.share(shareData);
} catch (error) {
if (!(error instanceof DOMException && error.name === "AbortError")) {
// Web Share APIを中断した場合以外の処理
}
}
All Code
When the Web Share API is available, it is used, and when it is not available, the link is made available as a copy to the clipboard as an alternative.
try {
const shareData = {
title: "MDN",
text: "Learn web development on MDN!",
url: "https://developer.mozilla.org",
};
const canWebShareApi =
!!navigator.share && navigator.canShare?.(shareData);
if (canWebShareApi) {
await navigator.share(shareData);
return;
}
await navigator.clipboard.writeText(url);
} catch (error) {
if (!(error instanceof DOMException && error.name === "AbortError")) {
...
}
}
Summary
It is nice to see that a little code can raise the user experience.