useContext is very useful for exchanging data between components. The implementation article for useContext is here.
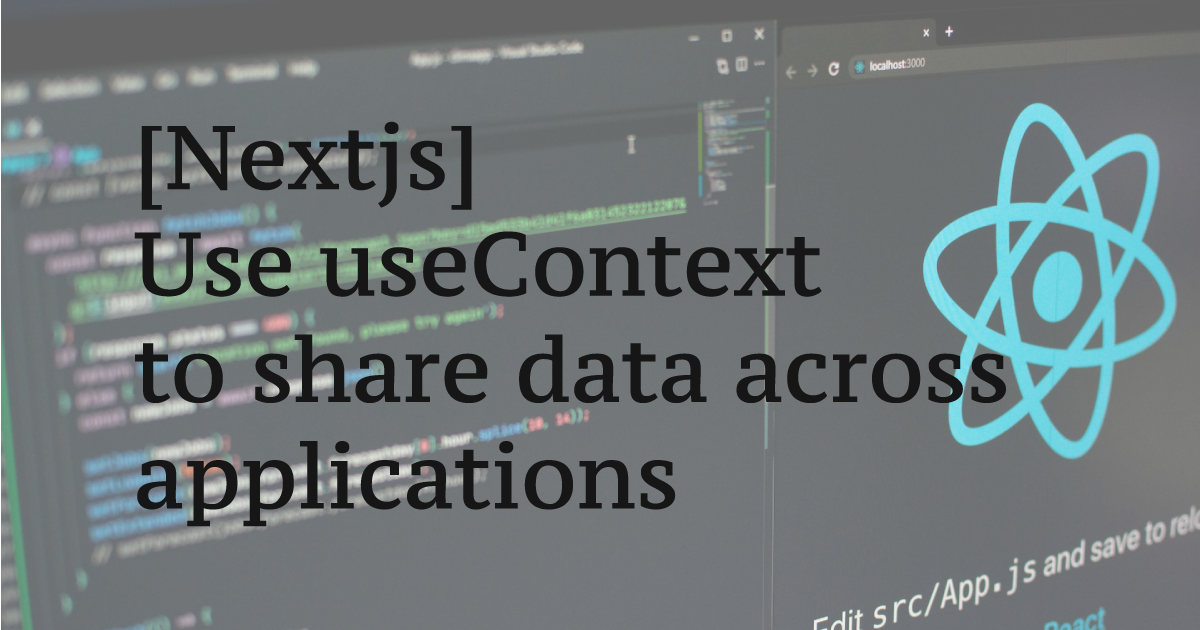
In the above article, the state is managed only by state, so the state is initialized when the browser is closed or reloaded. If you want to persist data even when the browser is closed, use localStorage.
What is Web Storage API?
This is a mechanism that allows the browser to save data as a key value pair. It is very simple, can only store string types, and only allows synchronous operations. These can be used through the Window object, and data can be saved, referenced, and deleted by creating a Storage instance. The maximum size of cookies is 4KB, but localStorage is also attractive because it can store up to 10MB of data. There are two types of Web Storage mechanisms.
sessionStorage
Save as page session. The data will continue to be retained even if the browser is reloaded. Discard data by closing the browser, closing the tab, etc.
localStorage
Your data persists even when you reload your browser, close your browser, or close tabs.
Official site
More details are provided in the official document. please refer to.
Save useContext state to localStorage
I added the localStorage implementation to the previous article where I implemented useContext. Now you can persist your data by saving it to localStorage.
Set the state and function to do the following in DataContext.Provider.
- data : save data between components
- setData : Update state and save to localStorage
- initData: initialize state and delete localStorage
Save to localStorage
Create a setData function. localStorage can be implemented simply with KeyValuePair.
You can save data by simply setting the key with window.localStorage.setItem and passing the string.
When you want to save an array or object, use JSON.stringify to convert it to a JSON string before saving it.
const storeData = (data: DataType[]): void => {
setData(data)
window.localStorage.setItem(storageDataKeyName, JSON.stringify(data))
}
Link the setData and storeData functions with the value of DataContext.Provider.
Delete from localStorage
Delete the target data by setting the key to localStorage.removeItem.
const initData = (): void => {
localStorage.removeItem(storageDataKeyName)
setData([])
}
Code
import { DataType, DataValuesType } from '@/types/Data'
import { createContext, useState, useContext, useEffect } from 'react'
const defaultProvider: DataValuesType = {
data: [],
setData: () => [],
initData: () => void 0
}
const DataContext = createContext(defaultProvider)
const storageDataKeyName = 'local_storage_data_key'
export function useDataContext() {
return useContext(DataContext)
}
export function DataProvider({ children }: { children: React.ReactNode }) {
const [data, setData] = useState<DataType[]>([])
const init = () => {
const storedData = window.localStorage.getItem(storageDataKeyName)!
if (storedData) {
setData(JSON.parse(storedData))
}
}
const initData = (): void => {
localStorage.removeItem(storageDataKeyName)
setData([])
}
const storeData = (data: DataType[]): void => {
setData(data)
window.localStorage.setItem(storageDataKeyName, JSON.stringify(data))
}
useEffect(() => {
init()
}, [])
const value = {
data,
setData: storeData,
initData: initData
}
return <DataContext.Provider value={value}>{children}</DataContext.Provider>
}
Summary
Using localStorage is very convenient and allows us to persist the state of useContext.
However, data saved in localStorage can be accessed from any JavaScript, and will remain forever unless you write deletion processing. After understanding the nature of LocalStorage and considering security, I think it is desirable to consider it as an option.