Validation was added because there was a requirement to prevent startup when the appropriate environment variables were not defined in the .env.
Once this is added, an error message will be displayed at startup, prompting the user to modify the .env.
Definition of .env.
Define any environment variables in .env.
NODE_ENV="development"
DATABASE_URL="<database-url>"
REDIS_EXPIRED=100
Create EnvValidator class
Create a validator class for the environment variables in .env.
In the EnvValidator class, define the same properties as the environment variables defined in .env.
Annotate each property with the type you want to validate.
Call the validate function and throw an error, the application will not be able to start.
import { plainToClass } from 'class-transformer';
import {
IsEnum,
IsNotEmpty,
IsNumber,
IsString,
validateSync,
} from 'class-validator';
enum NodeEnvEnum {
Development = 'development',
Staging = 'staging',
Production = 'production',
}
export class EnvValidator {
@IsEnum(NodeEnvEnum)
NODE_ENV: NodeEnvEnum;
@IsNotEmpty()
@IsString()
DATABASE_URL: string;
@IsNotEmpty()
@IsNumber()
REDIS_EXPIRED: number;
}
export function validate(config: Record<string, unknown>) {
const validatedConfig = plainToClass(EnvValidator, config, {
enableImplicitConversion: true,
});
const errors = validateSync(validatedConfig, {
skipMissingProperties: false,
});
if (errors.length > 0) {
throw new Error(errors.toString());
}
return validatedConfig;
}
Set in ConfigModule.
Set ConfigModule in app.module.ts to validate on startup.
import { validate } from './env.validation';
@Module({
imports: [
ConfigModule.forRoot({
validate,
}),
],
})
export class AppModule {}
Summary
When an environment variable is added during multi-person development and there is a sharing omission, an error is displayed at start-up, so it is good that there is less time to pursue the cause.
The formula is more detailed, so please read it.
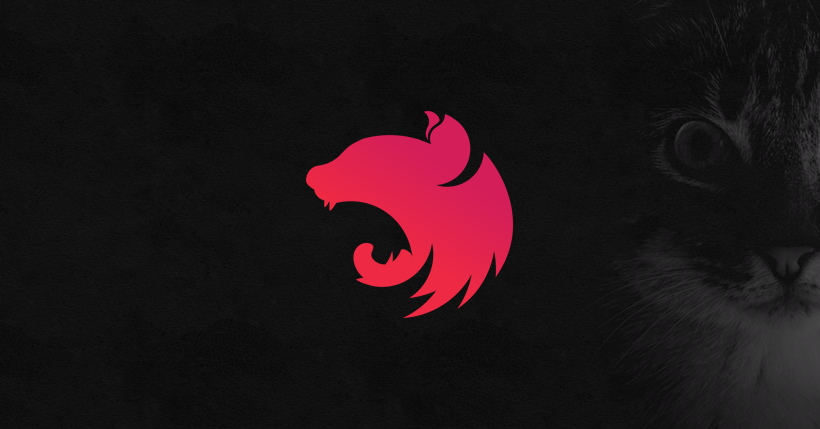