NestJS is an efficient and scalable Node.js application.
It also provides a generate command, which allows you to create an API simply and easily.
In this case, the Response returned from the API is converted into the intended format and returned.
The return format will look like this.
The data returned by the API is stored in result.
{
"status": "true",
"errorCode": "",
"result": []
}
目次
Response format conversion
Use Interceptors to convert Response data formats in common.
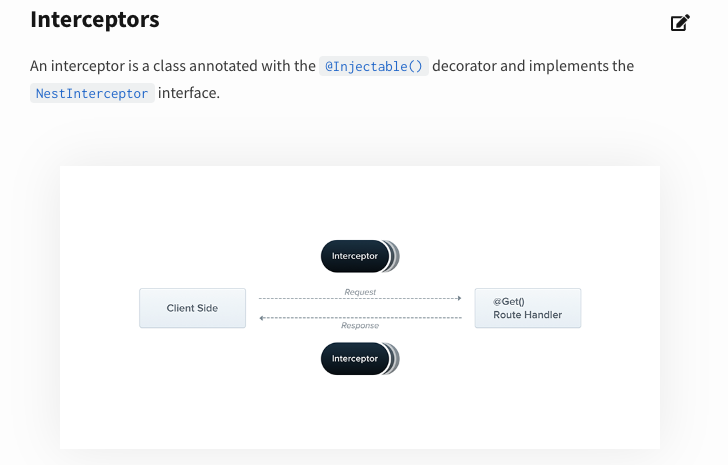
Specify the type with reference to the official website.
Define it as type Response and allow various types to be defined for the result.
Here, responseInterface.status = true;, but if the exception changes, set status=false in the ExceptionFilter.
This will determine whether the return from the API is 200 or otherwise.
import {
CallHandler,
ExecutionContext,
Injectable,
NestInterceptor,
} from '@nestjs/common';
import { Observable } from 'rxjs';
import { map } from 'rxjs/operators';
const responseInterface = {
status: false,
errorCode: '',
};
export type Response<T> = typeof responseInterface & {
result: T;
};
@Injectable()
export class TransformInterceptor<T>
implements NestInterceptor<T, Response<T>>
{
intercept(
context: ExecutionContext,
next: CallHandler,
): Observable<Response<T>> {
responseInterface.status = true;
return next
.handle()
.pipe(map((result) => Object.assign({}, responseInterface, { result })));
}
}
Binding interceptors
Load Interceptor in main.ts.
async function bootstrap() {
const app = await NestFactory.create(AppModule, { cors: true });
app.useGlobalInterceptors(new TransformInterceptor());
await app.listen(3000);
}
bootstrap();